# Introduction
Jazz² Resurrection is reimplementation of the game Jazz Jackrabbit 2 released in 1998. Supports various versions of the game (Shareware Demo, Holiday Hare '98, The Secret Files and Christmas Chronicles). Also, it partially supports some features of JJ2+ extension and MLLE. This project is hosted on GitHub.com/deathkiller/jazz2-native.
Jazz² Resurrection supports various versions of the original game, but it is recommended to use The Secret Files.
# Downloads
Latest version was released on May 6th, 2025 (61 days ago). Release notes can be found here.
Once you download and install Android version, you will be prompted with some security permissions to install app outside the Play Store.


# Preview
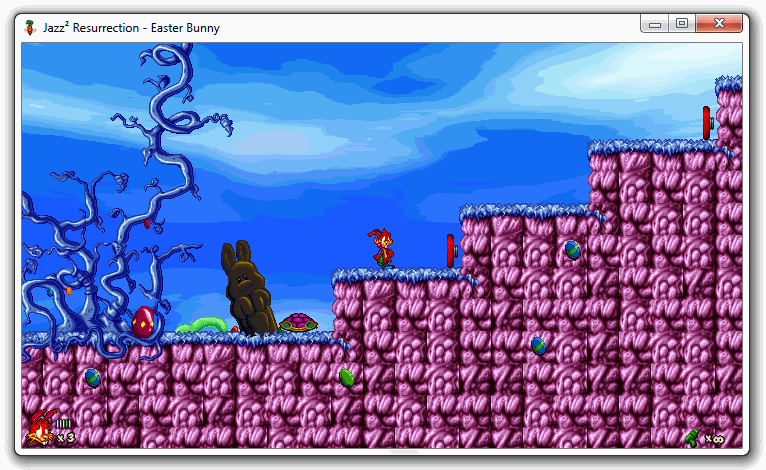
# Running the application
Windows
- Install Microsoft Visual C++ Redistributable
- Download the game
- Copy contents of original Jazz Jackrabbit 2 directory to
‹Game›\Source\
- Run
‹Game›\Jazz2.exe
,‹Game›\Jazz2_avx2.exe
or‹Game›\Jazz2_sdl2.exe
application
‹Game›
denotes path to Jazz² Resurrection. The game requires Windows 7 (or newer) and GPU with OpenGL 3.0 support. Game files should not be copied to Program Files
. Cache is recreated during intro cinematics on the first startup, so it can't be skipped.
Linux
- Download the game
- Install dependencies:
sudo apt install libcurl4 libglew2.2 libglfw3 libsdl2-2.0-0 libopenal1 libvorbisfile3 libopenmpt0
- Alternatively, install provided
.deb
or.rpm
package and dependencies should be installed automatically
- Alternatively, install provided
- Copy contents of original Jazz Jackrabbit 2 directory to
‹Game›/Source/
- If packages are used, the files must be copied to
~/.local/share/Jazz² Resurrection/Source/
or/usr/local/share/Jazz² Resurrection/Source/
instead, please follow instructions of specific package
- If packages are used, the files must be copied to
- Run
‹Game›/jazz2
or‹Game›/jazz2_sdl2
application- If packages are used, the game should be visible in application list
‹Game›
denotes path to Jazz² Resurrection. ~
denotes user's home directory. The game requires GPU with OpenGL 3.0 or OpenGL ES 3.0 (ARM) support. Cache is recreated during intro cinematics on the first startup, so it can't be skipped.
Alternatively, you can use package repository for your Linux distribution:
macOS
- Download the game and install provided
.dmg
application bundle - Copy contents of original Jazz Jackrabbit 2 directory to
~/Library/Application Support/Jazz² Resurrection/Source/
- Run the newly installed application
~
denotes user's home directory. Cache is recreated during intro cinematics on the first startup, so it can't be skipped.
Alternatively, you can install it using
brew install --cask jazz2-resurrection
Android
- Download the game
- Install
Jazz2.apk
orJazz2_x64.apk
on the device - Copy contents of original Jazz Jackrabbit 2 directory to
‹Storage›/Android/data/jazz2.resurrection/files/Source/
- On Android 11 or newer, you can Allow access to external storage in main menu, then you can use these additional paths:
‹Storage›/Games/Jazz² Resurrection/Source/
‹Storage›/Download/Jazz² Resurrection/Source/
- On Android 11 or newer, you can Allow access to external storage in main menu, then you can use these additional paths:
- Run the newly installed application
‹Storage›
usually denotes internal storage on your device. Content
directory is included directly in APK file, no action is needed. The game requires Android 5.0 (or newer) and GPU with OpenGL ES 3.0 support. Cache is recreated during intro cinematics on the first startup.
Nintendo Switch
- Download the game
- Install
Jazz2.nro
package (custom firmware is needed) - Copy contents of original Jazz Jackrabbit 2 directory to
/Games/Jazz2/Source/
on SD card - Run the newly installed application with enabled full RAM access
Cache is recreated during intro cinematics on the first startup, so it can't be skipped. It may take more time, so white screen could be shown longer than expected.
Web (Emscripten)
- Go to https://deat.tk/jazz2/wasm/
- Import episodes from original Jazz Jackrabbit 2 directory in main menu to unlock additional content
The game requires browser with WebAssembly and WebGL 2.0 support – usually any modern web browser.
Xbox (Universal Windows Platform)
- Download the game
- Install
Jazz2.cer
certificate if needed (the application is self-signed) - Install
Jazz2.msixbundle
package - Run the newly installed application
- Copy contents of original Jazz Jackrabbit 2 directory to destination shown in the main menu
- Alternatively, copy the files to
\Games\Jazz² Resurrection\Source\
on an external drive to preserve settings across installations, the application must be set toGame
type,exFAT
is recommended or correct read/write permissions must be assigned
- Alternatively, copy the files to
- Run the application again
# Building the application
This section contains only a brief explanation of the build process. For a more detailed explanation, including build configuration parameters, please refer to the developer documentation.
Windows
- Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option, then downloadBuild dependencies manually to
.\Libs\
- Can be disabled with
- Build the project with CMake
- Alternatively, download
Build dependencies to
.\Libs\
, open the solution in Microsoft Visual Studio 2019 (or newer) and build it
- Alternatively, download
Linux
- Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option, then downloadBuild dependencies manually to
./Libs/
- System libraries always have higher priority, there is no need to download them separately if your system already contains all dependencies
- In case of build errors, install following packages (or equivalent for your distribution):
libgl1-mesa-dev libglew-dev libglfw3-dev libsdl2-dev libopenal-dev libopenmpt-dev libcurl4-openssl-dev zlib1g-dev
- Can be disabled with
- Build the project with CMake
macOS
- Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option, then downloadBuild dependencies manually to
./Libs/
- Can be disabled with
- Build the project with CMake
Android
- Install Android SDK (preferably to
../android-sdk/
) - Install Android NDK (preferably to
../android-ndk/
) - Install Gradle (preferably to
../gradle/
) - Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option, then downloadBuild dependencies manually to
./Libs/
- Can be disabled with
- Build the project with CMake and
NCINE_BUILD_ANDROID
option
Nintendo Switch
- Install devkitPro toolchain
- Build the project with CMake and devkitPro toolchain
cmake -D CMAKE_TOOLCHAIN_FILE=${DEVKITPRO}/cmake/Switch.cmake -D NCINE_PREFERRED_BACKEND=SDL2
Web (Emscripten)
- Install Emscripten SDK (preferably to
../emsdk/
)cd .. git clone https://github.com/emscripten-core/emsdk.git cd emsdk ./emsdk install latest ./emsdk activate latest
- Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option
- Can be disabled with
- Copy required game files to
./Content/
directory – the files must be provided in advance - Build the project with CMake and Emscripten toolchain
Xbox (Universal Windows Platform)
- Build dependencies will be downloaded automatically by CMake
- Can be disabled with
NCINE_DOWNLOAD_DEPENDENCIES
option, then downloadBuild dependencies manually to
.\Libs\
- Can be disabled with
- Run CMake to create Microsoft Visual Studio 2019 (or newer) solution
cmake -D CMAKE_SYSTEM_NAME=WindowsStore -D CMAKE_SYSTEM_VERSION="10.0"
# License
This project is licensed under the terms of the GNU General Public License v3.0 and uses extensively modified nCine game engine.